Introduction to Shell Scripting in Ubuntu
Basics of Creating and Executing Shell Scripts to Automate Tasks

Introduction
Shell scripting is a powerful tool in the world of Linux, particularly in Ubuntu, as it allows users to automate repetitive tasks, manage system operations, and streamline workflows. Whether you're a beginner or an experienced developer, learning shell scripting can significantly enhance your productivity. This blog will guide you through the basics of creating and executing shell scripts in Ubuntu with practical code samples.
What is a shell script?
A shell script is a text file containing a series of commands that the shell (command line interpreter) can execute. Shell scripts are often used for automating tasks, simplifying complex sequences of commands, and managing system operations efficiently.
Why use shell scripts?
Automation: Automate repetitive tasks to save time.
Efficiency: Execute multiple commands in a single script.
Consistency: Ensure tasks are performed the same way every time.
Scheduling: Schedule scripts to run at specific times using tools like `cron`.
Getting Started
Creating Your First Shell Script
Let's start by creating a simple shell script. Open your terminal and follow these steps:
1. Open a Text Editor: You can use any text editor like `nano`, `vim`, or `gedit`. Here, we'll use `nano`.
nano my_first_script.sh
2. Write Your Script: Add the following lines to your script file:
#!/bin/bash
echo "Hello, World!"
3. Save and Exit: Save the file and exit the editor (for `nano`, press `Ctrl+X`, then `Y`, and `Enter`).
Making the Script Executable
Before you can run your script, you need to make it executable. Use the `chmod` command to change the file permissions:
chmod +x my_first_script.sh
1. Running Your Script
Now, you can execute your script by running:
./my_first_script.sh
You should see the output:
Hello, World!
Basic Shell Script Components
1. Shebang (`#!/bin/bash`)
The first line of the script (`#!/bin/bash`) is known as the shebang. It tells the system which interpreter to use to execute the script. In this case, it uses `bash`.
2. Comments
Comments are lines that are not executed by the shell. They are used to describe the code and are preceded by `#`.
# This is a comment
3. Variables
Variables are used to store data that can be referenced and manipulated within the script.
#!/bin/bash
greeting="Hello, World!"
echo $greeting
4. Conditional Statements
Conditional statements allow you to execute code based on certain conditions.
#!/bin/bash
number=10
if [ $number -gt 5 ]; then
echo "The number is greater than 5."
else
echo "The number is 5 or less."
fi
5. Loops
Loops allow you to repeat a block of code multiple times.
#!/bin/bash
for i in {1..5}
do
echo "Iteration $i"
done
6. Functions
Functions are reusable blocks of code that can be called with a single command.
#!/bin/bash
function greet() {
echo "Hello, $1!"
}
greet "Roger"
Practical Examples
1. Backup Script
Here's a simple script to back up a directory:
#!/bin/bash
SOURCE_DIR="/home/roger/Documents"
BACKUP_DIR="/home/roger/Backup"
# Create backup directory if it doesn't exist
mkdir -p $BACKUP_DIR
# Copy files to backup directory
cp -r $SOURCE_DIR/* $BACKUP_DIR/
echo "Backup completed successfully!"
2. Disk Usage Monitoring
This script checks the disk usage and sends an alert if it exceeds a certain threshold:
#!/bin/bash
THRESHOLD=80
DISK_USAGE=$(df / | grep / | awk '{ print $5 }' | sed 's/%//g')
if [ $DISK_USAGE -gt $THRESHOLD ]; then
echo "Warning: Disk usage is above $THRESHOLD%."
else
echo "Disk usage is within safe limits."
fi
3. Automated System Update
A script to automate system updates:
#!/bin/bash
echo "Updating system..."
sudo apt update && sudo apt upgrade -y
echo "System update completed!"
Conclusion
Shell scripting is an essential skill for anyone working with Linux systems, particularly in Ubuntu. By automating tasks, you can save time and reduce errors, making your workflow more efficient. This blog covered the basics of creating and executing shell scripts, including variables, conditional statements, loops, and functions. With these fundamentals, you can start exploring more advanced scripting techniques to further enhance your productivity.
Happy scripting!
What's Your Reaction?
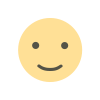
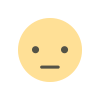
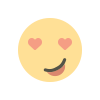
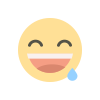
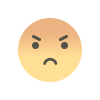
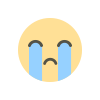
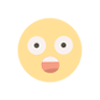